글
8장 구조체
[구조체]
- 구조체: 여러 개의 임의의 자료형의 값들의 모임을 나타내는 자료형
- 예) 여러 사람의 학번, 이름, 학과를 저장하는 변수 선언
구조체를 사용하지 않은 경우
int id1, id2, id3;
char name1[10], name2[10], name3[10];
int dept1, dept2, dept3
구조체를 사용한 경우
struct student{
int id;
char name[10];
int dept;
};
struct student p1, p2, p3;
[구조체 정의]
- 1. 구조체의 자료구조를 정의
- 2. 변수를 선언
- 구조체 정의
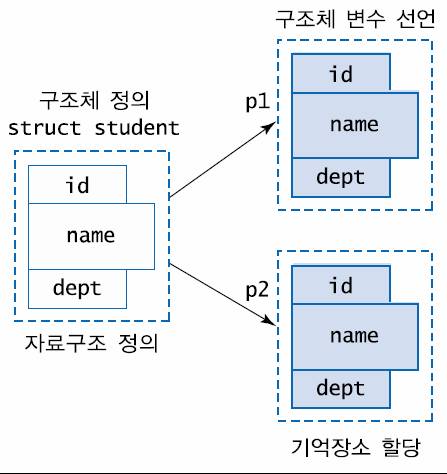
struct student{
int id;
char name[10];
int dept;
}; .... ' ; ' 빠뜨리지 않도록 주의
※ student 구조체 이름, 태그이름
id, name, dept 구조체 원소, 필드, 멤버
- 구조체 정의 후 변수 선언
struct student person;
struct student p1, p2;
- 구조체 정의와 함께 변수선언
struct student{
int id;
char name[10];
int dept;
}p1, p2;
[구조체 멤버 접근]
- 구조체 멤버 접근: 구조체변수.멤버이름(멤버 연산자.)
person.id person.name person.dept
- 예) person.id = 20030134 ... 학번 초기화
gets(person.name); ... 문자열 입력
scanf("%d", &person.dept); ... 학과코드 입력
[중첩된 구조체]
- 중첩된 구조체
구조체의 멤버로서 다른 구조체를 사용할 수 있음
struct triangle{
struct point.a;
struct point b;
struct point c;
};
[구조체의 초기화]
- 구조체의 초기화 : 배열과 같이 { }를 사용하여 서언과 함께 초기화 가능
struct test {
int a;
double f[2]; ...5개의 초기값이 필요
struct point p;
};
struct test s1 = {1, 2.1, 4.5 , 5, 7 };
struct test s2 = {1, 2.1, 4.5 , 5 }; … s2.p.y = 0
struct test t1 = { 1, {2.1, 4.5}, {5, 7} };
struct test t2 = { 1, {2.1}, {5, 7} }; … t2.f[1] = 0.0
초기값이 멤버 수 보다 적으면 나머지는 0으로 간주됨
[구조체의 치환과 비교]
- 구조체의 치환은 허용됨: 구조체의 모든 멤버들이 복사됨(배열은 치환 불가)
- 구조체의 비교는 허용되지 않음: 개별적으로 멤버 비교해야 함
struct point p1 = {4.0, 10.0};
struct point p2;
p2 = p1; /* 구조체 치환 */
printf("p2: x = %f, y = %f\n", p2.x, p2.y);
if (p1.x == p2.x && p1.y == p2.y) /* 구조체 비교: 멤버 별로 */
printf("두 점 p1과 p2는 같다.\n");
[구조체와 함수]
- 구조체는 함수의 인수 및 반환 값으로 사용 가능함
★ 인수 및 반환값은 복사되어 전달됨
구조체의 크기가 큰 경우에는 비효율적
예)
학생 데이타에 대한 구조체:
학번, 이름, 나이, 성적
struct student{
int sno;
char[30] name;
int age;
double gpa;
};
학생이 여러명일텐데, 배열을 선언해보자 (5명)
struct student boys[5] = {
{1012, Kim, 21, 3.45},{1009, Lee, 20, 3.91}, {1023, Park, 25, 2.92), (1030, Jung, 22, 3.14}, {1002, Choi, 20, 4.03}
};
Double compute_average(struct student pers[]);
다섯명의 학생의 평균을 구하라
void main(){
struct student boys[5] = {
{1012, Kim, 21, 3.45},{1009, Lee, 20, 3.91}, {1023, Park, 25, 2.92), (1030, Jung, 22, 3.14}, {1002, Choi, 20, 4.03}
};
double avg;
avg = compute_average(boys);
printf("average of 5 students = %5.2f \n", avg);
} //main.
Double compute_average(struct student pers[]){
double total = 0;
int i;
for(i=0;i<5;i++) total = total + per[i].gpa;
return total/5;
} // compute_average
=== 프로그래밍 ===
#include <stdio.h>
struct student{
int sno;
char name[30];
int age;
double gpa;
};
double compute_average(struct student pers[]);
int main(void){
double avg;
struct student boys[5] = {
{1012, "Kim", 21, 3.45}, {1009,"Lee", 20, 3.91}, {1023, "Park", 25, 2.92}, {1030, "Jung", 22, 3.14}, {1002, "Choi", 20, 4.03}
};
avg = compute_average(boys);
printf("average of 5 students = %5.2lf \n", avg);
return 0;
} //main.
double compute_average(struct student pers[])
{
double total = 0;
int i;
for(i=0;i<5;i++) total = total + pers[i].gpa;
return total/5;
} // compute_average
===
== 프로그램 ==
#include <stdio.h>
#include <string.h>
struct student{
int sno;
char name[30];
int age;
double gpa;
};
struct student find_student(struct student pers[], char na[]);
int main(void){
struct student boys[5] = {
{1012, "Kim", 21, 3.45}, {1009,"Lee", 20, 3.91}, {1023, "Park", 25, 2.92}, {1030, "Jung", 22, 3.14}, {1002, "Choi", 20, 4.03}
};
char aname[30];
struct student astu;
do{
printf("이름은? : ");
scanf("%s", aname);
astu = find_student (boys, aname);
if(aname[0] == '$') break;
printf("sno=%d age=%d gpa=%5.2f \n", astu.sno, astu.age, astu.gpa);
}while(1);
return 0;
} //main.
struct student find_student(struct student pers[], char na[])
{
int i;
for(i=0;i<5;i++)
if(strcmp(pers[i].name, na) == 0) return pers[i];
na[0] = '$';
return pers[0];
}
==
==과제==
위의 구문을 손봐서 만들기~....
학점을 받아서, 그 학점보다 더 잘 받은 사람을 찍어줘라...
학번 이름 나이 학점학점에 음수를 처주면 종료